A graphical user interface (GUI) is a type of user interface that allows users to interact with electronic devices through graphical elements such as icons, buttons, windows, and menus. It provides a visual representation of the system’s features and functions, making it easier for users to interact with the software or hardware.
GUIs offer a more intuitive and user-friendly approach compared to command-line interfaces, where users have to enter commands through text-based input. With GUIs, users can perform actions by simply clicking on buttons, selecting options from menus, or dragging and dropping elements on the screen.
Interactive input methods are an integral part of GUIs, allowing users to provide input to the system through various means. Some common interactive input methods in GUIs include:
- Mouse: Users can move the mouse pointer on the screen and click buttons to perform actions. The mouse is used for selecting items, dragging and dropping objects, and interacting with graphical elements.
- Keyboard: Although GUIs primarily rely on graphical elements, users can still input text or commands through the keyboard. This is useful for tasks such as typing in text fields, entering search queries, or executing keyboard shortcuts.
- Touchscreen: GUIs on mobile devices or tablets often incorporate touchscreens as an input method. Users can interact with the interface directly by tapping, swiping, or pinching on the screen.
- Stylus or Pen Input: Some touch-enabled devices support stylus or pen input, providing more precision and control for tasks such as drawing, handwriting recognition, or taking notes.
- Voice Recognition: Advanced GUIs may include voice recognition capabilities, allowing users to provide input through spoken commands. This input method can be particularly useful in hands-free or accessibility scenarios.
- Gestures: Certain GUIs support gesture recognition, where users can perform specific hand or finger movements to trigger actions. This input method is commonly used in touch-based interfaces, such as swiping across the screen or pinching to zoom.
These interactive input methods enhance the usability of GUIs by offering multiple ways for users to interact with the system, catering to different preferences and accessibility needs.
Windows and Icons
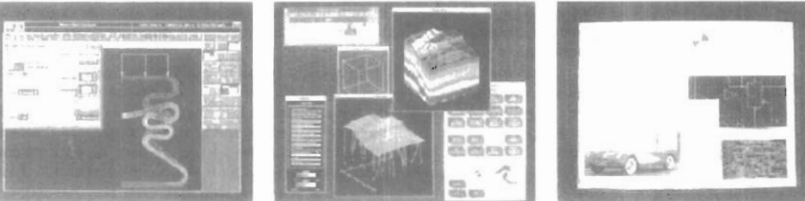
Windows and icons are fundamental elements of graphical user interfaces (GUIs), specifically referring to the visual representation of applications and files within an operating system environment. Let’s explore each of these elements:
- Windows: In GUIs, a window represents a graphical container that displays the content and functionality of an application or program. It is a rectangular area on the screen that can be resized, minimized, maximized, and moved around. Each window typically contains various graphical components such as menus, buttons, text fields, and images, allowing users to interact with the application’s features and perform tasks.
Windows provide a multitasking environment, enabling users to run multiple applications simultaneously and switch between them. They offer a convenient way to organize and manage different software and documents, as users can have separate windows for each application or file they are working on.
- Icons: Icons are small graphical representations or symbols that represent applications, files, folders, or system functions. They are often displayed on the desktop or in various locations within the GUI, such as the taskbar, start menu, or file manager.
Icons serve as visual shortcuts, allowing users to quickly access applications or files without navigating through complex folder structures. By clicking or tapping on an icon, the associated application or file is launched or opened. Icons can also provide visual cues or indications about the status or type of the represented item.
Users can customize icons by changing their appearance, size, or arrangement to suit their preferences or organizational needs. Additionally, icons may support drag-and-drop functionality, enabling users to move or copy files by dragging the corresponding icon to a desired location.
Windows and icons are key components of GUIs, working together to provide users with a visual representation of the system’s applications and files. They contribute to the overall usability and user-friendliness of the interface by allowing users to interact with their digital environment in an intuitive and visually appealing manner.
Here’s an example of a simple Windows and Icons program written in the C programming language:
#include <windows.h>
LRESULT CALLBACK WindowProcedure(HWND, UINT, WPARAM, LPARAM);
int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, LPSTR lpszCmdLine, int nCmdShow)
{
HWND hwnd;
MSG messages;
WNDCLASSEX wincl;
wincl.hInstance = hInstance;
wincl.lpszClassName = "Window";
wincl.lpfnWndProc = WindowProcedure;
wincl.style = CS_DBLCLKS;
wincl.cbSize = sizeof(WNDCLASSEX);
wincl.hIcon = LoadIcon(NULL, IDI_APPLICATION);
wincl.hIconSm = LoadIcon(NULL, IDI_APPLICATION);
wincl.hCursor = LoadCursor(NULL, IDC_ARROW);
wincl.lpszMenuName = NULL;
wincl.cbClsExtra = 0;
wincl.cbWndExtra = 0;
wincl.hbrBackground = (HBRUSH)COLOR_BACKGROUND;
if (!RegisterClassEx(&wincl))
return 0;
hwnd = CreateWindowEx(
0,
"Window",
"Windows and Icons",
WS_OVERLAPPEDWINDOW,
CW_USEDEFAULT,
CW_USEDEFAULT,
544,
375,
HWND_DESKTOP,
NULL,
hInstance,
NULL);
ShowWindow(hwnd, nCmdShow);
while (GetMessage(&messages, NULL, 0, 0))
{
TranslateMessage(&messages);
DispatchMessage(&messages);
}
return messages.wParam;
}
LRESULT CALLBACK WindowProcedure(HWND hwnd, UINT message, WPARAM wParam, LPARAM lParam)
{
switch (message)
{
case WM_DESTROY:
PostQuitMessage(0);
break;
default:
return DefWindowProc(hwnd, message, wParam, lParam);
}
return 0;
}
INPUT OF GRAPHICAL DATA
Graphical data input refers to the process of capturing or entering visual information into a computer system or software application. There are several methods and devices commonly used for inputting graphical data:
- Scanning: Scanners are devices that convert physical images or documents into digital format. They capture graphical data by scanning the image or document using sensors and creating a digital representation. Scanned images can be edited, stored, and processed on a computer.
- Digital Cameras: Digital cameras allow users to capture photographs or videos, which can be directly transferred to a computer or storage device. The images or videos captured can serve as graphical data that can be edited, analyzed, or incorporated into various applications.
- Drawing Tablets: Drawing tablets, also known as graphics tablets or pen tablets, enable users to draw or sketch directly on a specialized surface using a stylus or pen-like input device. The tablet detects the pen’s movements and pressure, converting them into digital graphical data that can be manipulated, edited, or used in digital art or design applications.
- Touchscreens: Touchscreen devices, such as smartphones, tablets, or interactive displays, allow users to directly interact with graphical elements on the screen using their fingers or a stylus. Users can draw, annotate, or manipulate graphical data by touching the screen, enabling intuitive input for various applications.
- Optical Character Recognition (OCR): OCR technology is used to convert printed or handwritten text into machine-readable digital text. OCR software scans and analyzes graphical data containing text, extracting the characters and converting them into editable and searchable text format.
- Computer-Aided Design (CAD) Input Devices: CAD applications often use specialized input devices like 3D mice or digitizing tablets. These devices allow precise input of graphical data for creating or editing three-dimensional models, architectural plans, or engineering drawings.
- Motion Capture: Motion capture technology records the movements of objects or individuals using sensors or cameras. It captures the motion as graphical data, which can be used for various applications such as animation, virtual reality, or biomechanical analysis.
These are just a few examples of how graphical data can be inputted into computer systems. The choice of input method depends on the specific requirements, application, and desired level of precision or detail needed for the graphical data.
INPUT FUNCTIONS
Input functions are an essential aspect of computer systems and software applications that enable users to provide data or instructions to the system. They allow users to interact with the computer and provide input that can be processed and utilized by the system. Here are some common input functions:
- Text Input: Users can enter text-based data using keyboards, keypads, or virtual keyboards on touchscreens. Text input is widely used for tasks such as typing in documents, filling out forms, entering commands, or providing textual information to applications.
- Mouse Input: A mouse is a pointing device that allows users to move a cursor or pointer on the screen. By clicking or dragging the mouse buttons, users can interact with graphical elements, select items, navigate menus, and perform various actions within software applications.
- Touch Input: Touchscreens on devices like smartphones, tablets, or interactive displays enable users to directly interact with the screen using their fingers or a stylus. Users can tap, swipe, pinch, or perform other gestures to provide input, navigate interfaces, select options, or interact with applications.
- Voice Input: Voice recognition technology allows users to provide input to computer systems using spoken commands or speech-to-text conversion. Users can dictate text, initiate actions, search for information, or control applications by speaking naturally to the system.
- Gesture Input: Gesture recognition enables users to provide input by making specific hand or body movements. This input method is commonly used in devices equipped with cameras or sensors that can detect and interpret gestures. Users can perform gestures like waving, swiping, or pinching to trigger actions or interact with applications.
- Sensor Input: Many modern devices are equipped with various sensors such as accelerometers, gyroscopes, GPS, or biometric sensors. These sensors provide input by capturing data about the device’s orientation, movement, location, or physical characteristics. Sensor input can be used in applications like gaming, fitness tracking, navigation, or authentication.
- File Input: Users can input data by selecting and opening files from their computer or external storage devices. This is commonly done through file dialogs or drag-and-drop functionality, allowing users to provide input by choosing specific files for processing or accessing their contents.
- Network Input: Users can input data or instructions to remote systems or online services over a network connection. This can include tasks like sending emails, submitting forms on websites, collaborating in real-time, or interacting with cloud-based applications.
These input functions enable users to provide a wide range of data and instructions to computer systems or software applications, allowing for interaction, control, and data entry in various contexts. The choice of input function depends on the device, application, and user preferences or requirements.
INTERACTIVE PICTURE-CONSTRUCTION TECHNIQUES
Interactive picture-construction techniques refer to methods or tools that allow users to create or modify pictures or graphics in a dynamic and interactive manner. These techniques provide users with real-time feedback and control over the construction or manipulation of visual elements. Here are some common interactive picture-construction techniques:
- Drawing Tools: Drawing tools provide users with the ability to create or modify pictures by manually drawing or sketching. These tools include various brushes, pens, pencils, and erasers that simulate real-world drawing instruments. Users can interactively apply strokes, shapes, colors, and textures to construct or edit pictures.
- Shape Manipulation: Interactive shape manipulation techniques enable users to create, transform, and manipulate geometric shapes within a picture. Users can interactively adjust parameters such as size, position, rotation, and proportions of shapes. Tools like resizing handles, rotation handles, and dragging points allow users to modify the shape’s appearance and position.
- Layering and Composition: Interactive picture-construction techniques often involve working with layers or composition tools. Layers allow users to organize elements of a picture into separate visual planes, enabling control over their visibility, stacking order, and interaction. Composition tools provide options for arranging, aligning, grouping, or overlapping elements within a picture to achieve desired visual compositions.
- Transformation Tools: Transformation tools allow users to interactively transform or distort selected portions of a picture. Users can apply operations such as scaling, rotation, skewing, flipping, or warping to modify the shape, perspective, or position of specific elements. These tools provide interactive handles or controls that users can manipulate to achieve the desired transformation.
- Interactive Filters and Effects: Picture-construction techniques often include interactive filters and effects that allow users to apply visual enhancements or modifications to the picture. Users can interactively adjust parameters such as brightness, contrast, saturation, blur, or color balance to create desired visual effects.
- Undo and Redo: Interactive picture-construction tools typically offer undo and redo functionality, enabling users to revert or repeat actions performed during the construction process. This feature provides flexibility and control, allowing users to experiment, correct mistakes, or iterate on their picture-construction process.
- Real-Time Preview: Many interactive picture-construction tools provide real-time preview capabilities, allowing users to see the immediate effect of their actions or modifications. This instant feedback enables users to make informed decisions and adjust their construction techniques accordingly.
These techniques empower users to actively participate in the creation or modification of pictures or graphics, providing a more engaging and dynamic experience. They enable users to have fine-grained control over visual elements and facilitate the exploration of different artistic or design possibilities.
VIRTUAL-REALITY ENVIRONMENTS
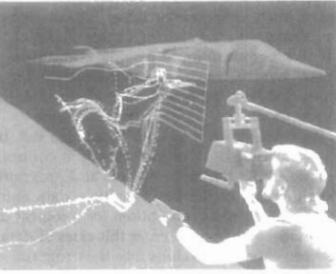
Virtual reality (VR) environments are computer-generated simulated worlds or experiences that aim to immerse users in a virtual three-dimensional environment. Users can interact with and explore these environments using specialized VR devices such as headsets and motion controllers. Here are key aspects and features of virtual reality environments:
- Head-Mounted Display (HMD): Users wear a head-mounted display (HMD), also known as a VR headset, which typically consists of a high-resolution screen for each eye. The headset tracks the user’s head movements and adjusts the displayed images accordingly, providing a sense of presence and immersion in the virtual environment.
- Motion Tracking: VR environments incorporate motion tracking systems to monitor the user’s movements and translate them into the virtual space. This enables users to walk, turn, and interact naturally within the virtual environment. Motion tracking technologies may use cameras, infrared sensors, or other tracking mechanisms to capture the user’s position and movements.
- Controllers and Input Devices: VR environments often employ handheld controllers or input devices to enable interaction within the virtual world. These devices can track the user’s hand movements, gestures, and button inputs, allowing users to manipulate objects, interact with the environment, and perform actions in a natural and intuitive way.
- Immersive 3D Graphics: Virtual reality environments prioritize realistic and immersive 3D graphics to create a sense of presence and believability. High-quality graphics, textures, lighting effects, and animations are used to enhance the realism and visual fidelity of the virtual environment, making it feel more immersive and engaging.
- Spatial Audio: Virtual reality environments often incorporate spatial audio technologies to provide an immersive audio experience. Sounds are positioned in 3D space to match the user’s location and orientation, creating a sense of depth and realism. This helps to enhance the overall immersion and presence within the virtual environment.
- Interactive Elements and Environments: VR environments are designed to provide interactive elements and environments that users can engage with. This can include objects that can be picked up, manipulated, or interacted with, as well as interactive elements within the environment such as buttons, switches, puzzles, or challenges. Users can explore, interact, and navigate within the virtual world to complete tasks or experience virtual scenarios.
- Multiplayer and Social Interaction: Virtual reality environments often support multiplayer functionality, enabling users to interact and collaborate with others in the same virtual space. This can involve real-time communication, cooperative tasks, competitive gameplay, or social interactions within the virtual environment.
Virtual reality environments offer immersive and interactive experiences that can be used for a variety of applications, including gaming, education, training, simulations, architectural visualization, and therapeutic interventions. By simulating realistic and interactive virtual worlds, VR environments aim to transport users to new experiences and provide a heightened sense of presence and engagement.