In computer graphics, points and lines are fundamental geometric primitives used to represent and create graphical elements on a digital display. They form the building blocks for more complex shapes and objects in a virtual environment.
POINTS in computer graphics
A point in computer graphics represents a single coordinate location in space. It is often defined by its x, y, and z coordinates in a three-dimensional (3D) space or by its x and y coordinates in a two-dimensional (2D) space. Points are typically used to represent vertices or endpoints of other graphical elements such as lines, curves, or polygons.
LINES in Computer graphics
A line is a straight path connecting two points. It can be defined by specifying the coordinates of its two endpoints. Lines can be 2D or 3D, depending on the dimensionality of the coordinate system being used. In 2D graphics, lines are typically represented as a series of pixels connected together to form a continuous path. In 3D graphics, lines can be rendered using algorithms such as Bresenham’s line algorithm or by utilizing mathematical equations like parametric or vector-based representations.
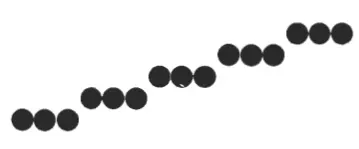
Points and lines are essential for creating various shapes and objects in computer graphics. By connecting multiple points with lines, complex structures such as polygons, curves, and three-dimensional objects can be constructed. Additionally, points and lines play a crucial role in rendering techniques like wireframe modeling, where objects are represented as a collection of interconnected lines.
Computer graphics systems provide APIs (Application Programming Interfaces) that allow programmers to manipulate points and lines through various operations such as translation, rotation, scaling, and clipping. These operations enable the creation and transformation of graphical elements, providing the basis for rendering realistic and interactive visuals in applications ranging from video games to architectural design software.
LINE-DRAWING ALGORITHMS
In computer graphics, line-drawing algorithms are methods used to generate a set of points that approximate a straight line between two given endpoints. These algorithms determine which pixels or coordinates should be illuminated or included to create the illusion of a continuous line.
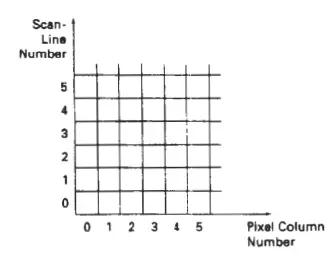
Here are some commonly used line-drawing algorithms:
- Digital Differential Analyzer (DDA) Algorithm: The DDA algorithm calculates the incremental change in x and y coordinates between two endpoints and uses these increments to determine the next pixel to be plotted. It works by dividing the total distance between endpoints into equal steps and incrementing the x and y coordinates accordingly. The algorithm is relatively simple but can introduce rounding errors.
- Bresenham’s Line Algorithm: Bresenham’s algorithm is an efficient method for drawing lines on a raster display. It uses integer arithmetic and avoids floating-point calculations, making it computationally faster. The algorithm determines which pixel to plot based on the decision variable, which is calculated using the difference between the actual line position and the ideal line position at each step. Bresenham’s algorithm is widely used due to its simplicity and speed.
- Midpoint Line Algorithm: The midpoint line algorithm, also known as the midpoint circle algorithm, can be extended to draw lines. It calculates an initial decision parameter based on the midpoint of the line and determines whether to move to the next pixel vertically or diagonally. The algorithm takes advantage of symmetry to reduce the number of calculations required. It is particularly useful for drawing lines with slopes close to 1 or -1.
- Xiaolin Wu’s Line Algorithm: Xiaolin Wu’s algorithm is an extension of Bresenham’s algorithm that provides anti-aliasing capabilities, allowing for smoother and more visually appealing lines. The algorithm takes advantage of the properties of the display’s color resolution to create the illusion of varying line thickness and smooth gradients along the line.
Digital Differential Analyzer (DDA) Algorithm
The Digital Differential Analyzer (DDA) algorithm is a straightforward method for drawing lines in computer graphics. It approximates a line by calculating the incremental change in x and y coordinates between two endpoints and plotting pixels along the line.
Here is how the DDA algorithm works:
- Calculate the differences between the x and y coordinates of the two endpoints: dx = x2 – x1 dy = y2 – y1
- Determine the number of steps required to increment from one endpoint to the other. This can be based on either the difference in the x or y coordinates, depending on which one has a larger magnitude. Let’s assume dx has the larger magnitude: steps = abs(dx)
- Calculate the increments for x and y: x_increment = dx / steps y_increment = dy / steps
- Set the initial point (x, y) to (x1, y1).
- Repeat the following steps ‘steps’ number of times:
- Plot the pixel at (round(x), round(y)).
- Increment x by x_increment.
- Increment y by y_increment.
The DDA algorithm uses the concept of linear interpolation to calculate the incremental changes in the x and y coordinates. By dividing the total distance between the endpoints into equal steps, it ensures that the line is evenly approximated. However, it is worth noting that the algorithm introduces rounding errors, which can lead to slight deviations from the exact line.
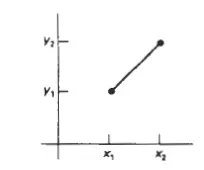
The DDA algorithm is relatively simple and easy to implement. However, it is not as efficient as some other line-drawing algorithms, especially when dealing with lines with steep slopes or near-horizontal lines. In such cases, Bresenham’s algorithm or other specialized algorithms may offer better performance.
Here’s an example of implementing the Digital Differential Analyzer (DDA) algorithm in C to draw a line:
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
void drawLineDDA(int x1, int y1, int x2, int y2) {
int dx = x2 - x1;
int dy = y2 - y1;
int steps;
// Determine the number of steps
if (abs(dx) > abs(dy)) {
steps = abs(dx);
} else {
steps = abs(dy);
}
// Calculate increments for x and y
float x_increment = (float)dx / steps;
float y_increment = (float)dy / steps;
// Set initial point
float x = x1;
float y = y1;
// Plot pixels along the line
for (int i = 0; i <= steps; i++) {
printf("Pixel at (%d, %d)\n", (int)round(x), (int)round(y));
x += x_increment;
y += y_increment;
}
}
int main() {
// Example usage
int x1 = 2, y1 = 3;
int x2 = 8, y2 = 9;
printf("Drawing line using DDA algorithm:\n");
drawLineDDA(x1, y1, x2, y2);
return 0;
}
Bresenham’s Line Algorithm
Bresenham’s Line Algorithm is an efficient method for approximating and rasterizing lines on a computer screen or display. It uses integer arithmetic and avoids costly floating-point calculations, making it computationally faster compared to other algorithms.
The algorithm works by determining the best pixels to plot along the line based on a decision variable. Here’s how it generally works:
- Given two endpoints, (x1, y1) and (x2, y2), calculate the differences in x and y coordinates: dx = x2 – x1 dy = y2 – y1
- Determine the increments for x and y based on the sign of dx and dy:
- If dx is positive, x_increment = 1; otherwise, x_increment = -1.
- If dy is positive, y_increment = 1; otherwise, y_increment = -1.
- Calculate the absolute values of dx and dy: abs_dx = absolute value of dx abs_dy = absolute value of dy
- Determine the initial decision parameter, which is based on the absolute differences in x and y: decision_param = 2 * abs_dy – abs_dx
- Set the initial point (x, y) to (x1, y1).
- Begin the iteration:
- Plot the pixel at (x, y).
- If the decision_param is greater than or equal to 0, increment y by y_increment and adjust the decision_param accordingly:
- y += y_increment
- decision_param -= 2 * abs_dx
- Increment x by x_increment and adjust the decision_param:
- x += x_increment
- decision_param += 2 * abs_dy
- Repeat the iteration until reaching the final endpoint (x2, y2).
By carefully selecting which pixel to plot at each step, Bresenham’s algorithm ensures that the line is approximated accurately while minimizing computational overhead. The decision parameter is used to determine whether to increment the y-coordinate or not at each step. The algorithm takes advantage of integer arithmetic and simple comparisons, making it efficient for line-drawing operations.
Bresenham’s Line Algorithm is widely used in computer graphics applications for drawing lines, wireframes, and other geometric shapes. It has applications in various fields, including computer-aided design (CAD), computer games, image processing, and more.
Bresenham’s Line Algorithm in C
Here’s an example of implementing Bresenham’s Line Algorithm in C to draw a line:
#include <stdio.h>
#include <stdlib.h>
void drawLineBresenham(int x1, int y1, int x2, int y2) {
int dx = abs(x2 - x1);
int dy = abs(y2 - y1);
int x_increment = (x1 < x2) ? 1 : -1;
int y_increment = (y1 < y2) ? 1 : -1;
int error = dx - dy;
while (x1 != x2 || y1 != y2) {
printf("Pixel at (%d, %d)\n", x1, y1);
int error2 = 2 * error;
if (error2 > -dy) {
error -= dy;
x1 += x_increment;
}
if (error2 < dx) {
error += dx;
y1 += y_increment;
}
}
printf("Pixel at (%d, %d)\n", x2, y2); // Print the last pixel
}
int main() {
// Example usage
int x1 = 2, y1 = 3;
int x2 = 8, y2 = 9;
printf("Drawing line using Bresenham's algorithm:\n");
drawLineBresenham(x1, y1, x2, y2);
return 0;
}
Midpoint Line Algorithm
The Midpoint Line Algorithm works by taking advantage of symmetry and incremental calculations. Here’s how it generally works:
- Given two endpoints, (x1, y1) and (x2, y2), calculate the differences in x and y coordinates: dx = x2 – x1 dy = y2 – y1
- Determine the increments for x and y based on the sign of dx and dy:
- If dx is positive, x_increment = 1; otherwise, x_increment = -1.
- If dy is positive, y_increment = 1; otherwise, y_increment = -1.
- Calculate the absolute values of dx and dy: abs_dx = absolute value of dx abs_dy = absolute value of dy
- Calculate the initial decision parameter based on the midpoint of the line: initial_decision = 2 * abs_dy – abs_dx
- Set the initial point (x, y) to (x1, y1).
- Begin the iteration:
- Plot the pixel at (x, y).
- If the decision parameter is greater than or equal to 0, adjust the y-coordinate and update the decision parameter:
- y += y_increment
- decision_param -= 2 * abs_dx
- Increment the x-coordinate and update the decision parameter:
- x += x_increment
- decision_param += 2 * abs_dy
By calculating the midpoint of the line and updating the decision parameter at each step, the algorithm determines which pixel to plot and moves along the line in a way that avoids floating-point calculations. It efficiently handles lines with slopes close to 1 or -1, and the use of integer arithmetic makes it fast and suitable for real-time rendering.
The Midpoint Line Algorithm can be extended from the Midpoint Circle Algorithm, which is used to draw circles. By drawing lines between the octants of a circle, lines with arbitrary slopes can be rasterized using this algorithm.
The Midpoint Line Algorithm is widely used in computer graphics applications for drawing lines, wireframes, and other geometric shapes. It provides an efficient way to rasterize lines and is often used in graphics libraries and rendering pipelines.
Midpoint Line Algorithm in C
#include <stdio.h>
#include <stdlib.h>
void drawLineMidpoint(int x1, int y1, int x2, int y2) {
int dx = abs(x2 - x1);
int dy = abs(y2 - y1);
int x_increment = (x1 < x2) ? 1 : -1;
int y_increment = (y1 < y2) ? 1 : -1;
int decision_param = 2 * dy - dx;
int x = x1;
int y = y1;
while (x != x2 || y != y2) {
printf("Pixel at (%d, %d)\n", x, y);
if (decision_param >= 0) {
y += y_increment;
decision_param -= 2 * dx;
}
x += x_increment;
decision_param += 2 * dy;
}
printf("Pixel at (%d, %d)\n", x2, y2); // Print the last pixel
}
int main() {
// Example usage
int x1 = 2, y1 = 3;
int x2 = 8, y2 = 9;
printf("Drawing line using Midpoint algorithm:\n");
drawLineMidpoint(x1, y1, x2, y2);
return 0;
}
Xiaolin Wu’s Line Algorithm
Xiaolin Wu’s Line Algorithm, also known as the Wu’s Line Algorithm, is an advanced line-drawing algorithm that provides anti-aliasing capabilities to render smoother and more visually appealing lines on a computer screen or display. It is an extension of Bresenham’s Line Algorithm.
Wu’s Line Algorithm works by taking advantage of the varying intensities of pixels along a line to create the illusion of a smooth line. Here’s how it generally works:
- Given two endpoints, (x1, y1) and (x2, y2), calculate the differences in x and y coordinates: dx = x2 – x1 dy = y2 – y1
- Determine the increments for x and y based on the sign of dx and dy, similar to other line-drawing algorithms.
- Calculate the absolute values of dx and dy, as well as the slope of the line: abs_dx = absolute value of dx abs_dy = absolute value of dy slope = dy / dx
- Determine the major axis (x or y) based on the absolute differences:
- If abs_dx is greater than abs_dy, the x-axis is the major axis.
- Otherwise, the y-axis is the major axis.
- Iterate along the major axis and perform the following steps:
- Calculate the intensity of the two pixels adjacent to the line at each step based on the fractional part of the coordinates.
- Plot the pixels with their respective intensities, considering both the foreground color and the background color.
- For the pixels adjacent to the line, calculate the alpha value (opacity) based on the fractional part of the coordinates and blend the colors accordingly to achieve anti-aliasing.
By considering the fractional part of the coordinates and blending the colors, Xiaolin Wu’s algorithm produces smoother lines by simulating gradual transitions from the line to the background. This results in better visual quality and reduces the “jagged” appearance often seen with other line-drawing algorithms.
Xiaolin Wu’s Line Algorithm is particularly useful when drawing lines at angles close to horizontal or vertical, as well as when dealing with high-resolution displays or rendering contexts where anti-aliasing is desired.
Xiaolin Wu’s Line Algorithm in C
Here’s an example of implementing Xiaolin Wu’s Line Algorithm in C to draw anti-aliased lines:
#include <stdio.h>
#include <math.h>
// Function to blend two colors based on alpha value
unsigned int blendColors(unsigned int color1, unsigned int color2, float alpha) {
unsigned int red1 = (color1 >> 16) & 0xFF;
unsigned int green1 = (color1 >> 8) & 0xFF;
unsigned int blue1 = color1 & 0xFF;
unsigned int red2 = (color2 >> 16) & 0xFF;
unsigned int green2 = (color2 >> 8) & 0xFF;
unsigned int blue2 = color2 & 0xFF;
unsigned int blendedRed = (1 - alpha) * red1 + alpha * red2;
unsigned int blendedGreen = (1 - alpha) * green1 + alpha * green2;
unsigned int blendedBlue = (1 - alpha) * blue1 + alpha * blue2;
return (blendedRed << 16) | (blendedGreen << 8) | blendedBlue;
}
// Function to draw an anti-aliased line using Xiaolin Wu's algorithm
void drawLineWu(int x1, int y1, int x2, int y2, unsigned int foregroundColor, unsigned int backgroundColor) {
int dx = x2 - x1;
int dy = y2 - y1;
float slope = (float)dy / dx;
int isSteep = abs(dy) > abs(dx);
if (isSteep) {
int temp = x1;
x1 = y1;
y1 = temp;
temp = x2;
x2 = y2;
y2 = temp;
}
if (x1 > x2) {
int temp = x1;
x1 = x2;
x2 = temp;
temp = y1;
y1 = y2;
y2 = temp;
}
dx = x2 - x1;
dy = y2 - y1;
slope = (float)dy / dx;
float y = y1;
for (int x = x1; x <= x2; x++) {
float intensity = y - floor(y);
unsigned int color1 = blendColors(foregroundColor, backgroundColor, 1 - intensity);
unsigned int color2 = blendColors(foregroundColor, backgroundColor, intensity);
if (isSteep) {
printf("Pixel at (%d, %d) with intensity %f\n", (int)y, x, intensity);
} else {
printf("Pixel at (%d, %d) with intensity %f\n", x, (int)y, intensity);
}
y += slope;
}
}
int main() {
// Example usage
int x1 = 2, y1 = 3;
int x2 = 8, y2 = 9;
unsigned int foregroundColor = 0xFF0000; // Red
unsigned int backgroundColor = 0x000000; // Black
printf("Drawing line using Xiaolin Wu's algorithm:\n");
drawLineWu(x1, y1, x2, y2, foregroundColor, backgroundColor);
return 0;
}
The drawLineWu
function takes the coordinates of two endpoints (x1, y1) and (x2, y2), as well as the foreground and background colors as parameters. The algorithm begins by calculating the differences in x and y, as well as the slope of the line.
Next, it checks if the line is steep (where the change in y is greater than the change in x) and if so, it swaps the x and y coordinates. It also checks if the line is going from right to left, and if so, it swaps the coordinates accordingly.
The algorithm then recalculates the differences and slope after the coordinate swaps.
The function iterates along the major axis (x or y) and calculates the intensity of the two pixels adjacent to the line at each step based on the fractional part of the y-coordinate. It uses the blendColors
function to blend the foreground and background colors based on the calculated intensity.
Inside the loop, the algorithm prints the coordinates of the pixel and the calculated intensity. The isSteep
flag is used to determine whether to print the coordinates as (y, x) or (x, y) to handle the coordinate swaps.
In the main
function, we provide example values for the endpoints, foreground color (red), and background color (black). We then call the drawLineWu
function to draw the anti-aliased line. The output will display the coordinates of the pixels along the line and their corresponding intensities.
Please note that this example assumes the coordinates and colors are integer values. If you want to handle non-integer coordinates or use different color representations, you may need to modify the code accordingly.
Parallel Line Algorithms
Parallel Line Algorithms are a group of algorithms used in computer graphics to efficiently draw multiple lines in parallel. These algorithms allow for the simultaneous rendering of multiple lines without repeating unnecessary calculations for each line. Here are two commonly used parallel line algorithms:
- DDA Parallel Line Algorithm: The Digital Differential Analyzer (DDA) Parallel Line Algorithm works by calculating the increments for x and y coordinates based on the differences between the endpoints of each line. The algorithm takes advantage of the fact that lines with the same slope can share the same incremental calculations. The steps to implement the DDA Parallel Line Algorithm are as follows:
- Calculate the differences in x and y coordinates for each line.
- Determine the maximum absolute difference (either dx or dy) among all the lines.
- For each line, calculate the increments for x and y based on the maximum difference.
- Iterate along the major axis (x or y) and increment the coordinates of each line accordingly.
- Plot the pixels for each line simultaneously.
- Bresenham Parallel Line Algorithm: The Bresenham Parallel Line Algorithm is an extension of Bresenham’s Line Algorithm, specifically designed for parallel line drawing. It utilizes the concept of Bresenham’s algorithm by taking advantage of integer arithmetic and incremental calculations. The steps to implement the Bresenham Parallel Line Algorithm are as follows:
- Calculate the differences in x and y coordinates for each line.
- Determine the maximum absolute difference (either dx or dy) among all the lines.
- For each line, calculate the increments for x and y based on the maximum difference.
- Iterate along the major axis (x or y) and increment the coordinates of each line accordingly.
- Use Bresenham’s algorithm to determine the pixels to plot for each line simultaneously.
Both the DDA Parallel Line Algorithm and the Bresenham Parallel Line Algorithm optimize the process of drawing multiple lines in parallel, reducing redundant calculations and improving rendering speed. These algorithms are commonly used in computer graphics applications where the simultaneous rendering of multiple lines is required, such as in CAD software, real-time graphics engines, and vector graphics editors.
Here’s an example of implementing the DDA Parallel Line Algorithm and the Bresenham Parallel Line Algorithm in C to draw multiple lines in parallel:
#include <stdio.h>
#include <stdlib.h>
// DDA Parallel Line Algorithm
void drawLinesDDA(int* x1, int* y1, int* x2, int* y2, int numLines) {
int maxDifference = 0;
for (int i = 0; i < numLines; i++) {
int dx = abs(x2[i] - x1[i]);
int dy = abs(y2[i] - y1[i]);
int difference = (dx > dy) ? dx : dy;
if (difference > maxDifference) {
maxDifference = difference;
}
}
for (int i = 0; i < maxDifference; i++) {
for (int j = 0; j < numLines; j++) {
if (i < maxDifference) {
float xIncrement = (float)(x2[j] - x1[j]) / maxDifference;
float yIncrement = (float)(y2[j] - y1[j]) / maxDifference;
int x = x1[j] + (int)(xIncrement * i + 0.5);
int y = y1[j] + (int)(yIncrement * i + 0.5);
printf("Pixel at (%d, %d) for line %d\n", x, y, j + 1);
}
}
}
}
// Bresenham Parallel Line Algorithm
void drawLinesBresenham(int* x1, int* y1, int* x2, int* y2, int numLines) {
int maxDifference = 0;
for (int i = 0; i < numLines; i++) {
int dx = abs(x2[i] - x1[i]);
int dy = abs(y2[i] - y1[i]);
int difference = (dx > dy) ? dx : dy;
if (difference > maxDifference) {
maxDifference = difference;
}
}
for (int i = 0; i < maxDifference; i++) {
for (int j = 0; j < numLines; j++) {
if (i < maxDifference) {
int dx = x2[j] - x1[j];
int dy = y2[j] - y1[j];
int xIncrement = dx / maxDifference;
int yIncrement = dy / maxDifference;
int x = x1[j] + (xIncrement * i);
int y = y1[j] + (yIncrement * i);
printf("Pixel at (%d, %d) for line %d\n", x, y, j + 1);
}
}
}
}
int main() {
// Example usage
int numLines = 3;
int x1[] = { 2, 4, 6 };
int y1[] = { 3, 5, 7 };
int x2[] = { 8, 10, 12 };
int y2[] = { 9, 11, 13 };
printf("Drawing lines using DDA Parallel Line Algorithm:\n");
drawLinesDDA(x1, y1, x2, y2, numLines);
printf("\nDrawing lines using Bresenham Parallel Line Algorithm:\n");
drawLinesBresenham(x1, y1, x2, y2, numLines);
return 0;
}
1 thought on “POINTS AND LINES in Computer graphics”