In computer graphics, the line function is a mathematical representation used to describe a straight line. It provides a way to determine the position of points along the line based on their coordinates and the equation of the line.
The general form of the line function is expressed as:
y = mx + b
Here, ‘m’ represents the slope of the line, which determines its steepness or inclination. The slope indicates how much the y-coordinate changes for every unit increase in the x-coordinate. A positive slope means the line rises from left to right, while a negative slope means the line falls from left to right.
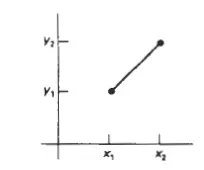
‘b’ is the y-intercept of the line, which represents the point where the line intersects the y-axis. It indicates the y-coordinate when x is equal to zero.
To use the line function, you can provide the x-coordinate of a point, and by substituting it into the equation, you can calculate the corresponding y-coordinate. This process allows you to generate a set of points that lie on the line.
By utilizing the line function, various operations can be performed on lines, such as:
- Determining if a point lies on the line: You can substitute the x and y coordinates of the point into the line equation and check if the equation holds true.
- Finding the intersection point of two lines: By solving the equations of two lines simultaneously, you can find the coordinates where the two lines intersect.
- Generating a set of points to draw the line: By incrementing the x-coordinate and calculating the corresponding y-coordinate using the line function, you can generate a series of points that can be used to draw the line on the screen.
The line function forms the basis for many line-drawing algorithms in computer graphics, such as the Digital Differential Analyzer (DDA), Bresenham’s algorithm, and midpoint algorithm. These algorithms optimize the computation and incrementally calculate points along the line to efficiently draw it on the screen.
By utilizing the line function and its associated algorithms, lines can be accurately represented and rendered in computer graphics applications, forming the foundation for creating shapes, curves, and complex images.
An example of a C program that demonstrates how to use the line function to generate points on a line
#include <stdio.h>
// Function to calculate points on a line using the line equation
void calculateLinePoints(int x1, int y1, int x2, int y2) {
int dx = x2 - x1;
int dy = y2 - y1;
// Calculate the slope (m)
float slope = (float)dy / dx;
// Calculate the y-intercept (b)
float yIntercept = y1 - slope * x1;
// Determine the range of x-coordinates
int startX = (x1 < x2) ? x1 : x2;
int endX = (x1 > x2) ? x1 : x2;
// Calculate and print the points on the line
for (int x = startX; x <= endX; x++) {
int y = slope * x + yIntercept;
printf("Point at (%d, %d)\n", x, y);
}
}
int main() {
// Example usage
int x1 = 2, y1 = 3;
int x2 = 8, y2 = 9;
printf("Generating points on the line:\n");
calculateLinePoints(x1, y1, x2, y2);
return 0;
}
In this program, the calculateLinePoints
the function takes the coordinates of two points (x1, y1)
and (x2, y2)
as input. It calculates the slope of the line using the line equation and then determines the y-intercept. By iterating through the range of x-coordinates, it calculates the corresponding y-coordinates using the line equation and prints the points on the line.
The main
function demonstrates an example usage by providing the coordinates (2, 3)
and (8, 9)
to generate points on the line connecting these two points. The calculated points are then printed to the console.