Complete Source code to make Quiz game in Android using java
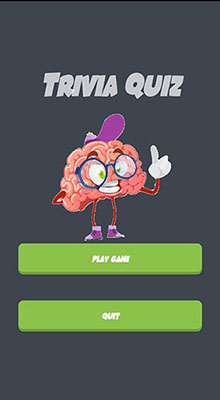
GameWon.java
package sarveshchavan777.triviaquiz;
import android.app.Activity;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
public class GameWon extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.game_won);
}
//This is onclick listener for button
//it will navigate from this activity to MainGameActivity
public void PlayAgain(View view) {
Intent intent = new Intent(GameWon.this, MainGameActivity.class);
startActivity(intent);
finish();
}
}
HomeScreen.java
package sarveshchavan777.triviaquiz;
import android.content.Intent;
import android.graphics.Typeface;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.TextView;
import info.hoang8f.widget.FButton;
public class HomeScreen extends AppCompatActivity {
FButton playGame,quit;
TextView tQ;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_home_screen);
//the below method will initialize views
initViews();
//PlayGame button - it will take you to the MainGameActivity
playGame.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
Intent intent = new Intent(HomeScreen.this,MainGameActivity.class);
startActivity(intent);
finish();
}
});
//Quit button - This will quit the game
quit.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
finish();
}
});
}
private void initViews() {
//initialize views here
playGame =(FButton)findViewById(R.id.playGame);
quit = (FButton) findViewById(R.id.quit);
tQ = (TextView)findViewById(R.id.tQ);
//Typeface - this is for fonts style
Typeface typeface = Typeface.createFromAsset(getAssets(),"fonts/shablagooital.ttf");
playGame.setTypeface(typeface);
quit.setTypeface(typeface);
tQ.setTypeface(typeface);
}
}
MainGameActivity.java
package sarveshchavan777.triviaquiz;
import android.app.Dialog;
import android.content.Intent;
import android.graphics.Color;
import android.graphics.Typeface;
import android.graphics.drawable.ColorDrawable;
import android.os.CountDownTimer;
import android.support.v4.content.ContextCompat;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.view.Window;
import android.widget.TextView;
import java.util.Collections;
import java.util.List;
import info.hoang8f.widget.FButton;
public class MainGameActivity extends AppCompatActivity {
FButton buttonA, buttonB, buttonC, buttonD;
TextView questionText, triviaQuizText, timeText, resultText, coinText;
TriviaQuizHelper triviaQuizHelper;
TriviaQuestion currentQuestion;
List<TriviaQuestion> list;
int qid = 0;
int timeValue = 20;
int coinValue = 0;
CountDownTimer countDownTimer;
Typeface tb, sb;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_game_main);
//Initializing variables
questionText = (TextView) findViewById(R.id.triviaQuestion);
buttonA = (FButton) findViewById(R.id.buttonA);
buttonB = (FButton) findViewById(R.id.buttonB);
buttonC = (FButton) findViewById(R.id.buttonC);
buttonD = (FButton) findViewById(R.id.buttonD);
triviaQuizText = (TextView) findViewById(R.id.triviaQuizText);
timeText = (TextView) findViewById(R.id.timeText);
resultText = (TextView) findViewById(R.id.resultText);
coinText = (TextView) findViewById(R.id.coinText);
//Setting typefaces for textview and buttons - this will give stylish fonts on textview and button etc
tb = Typeface.createFromAsset(getAssets(), "fonts/TitilliumWeb-Bold.ttf");
sb = Typeface.createFromAsset(getAssets(), "fonts/shablagooital.ttf");
triviaQuizText.setTypeface(sb);
questionText.setTypeface(tb);
buttonA.setTypeface(tb);
buttonB.setTypeface(tb);
buttonC.setTypeface(tb);
buttonD.setTypeface(tb);
timeText.setTypeface(tb);
resultText.setTypeface(sb);
coinText.setTypeface(tb);
//Our database helper class
triviaQuizHelper = new TriviaQuizHelper(this);
//Make db writable
triviaQuizHelper.getWritableDatabase();
//It will check if the ques,options are already added in table or not
//If they are not added then the getAllOfTheQuestions() will return a list of size zero
if (triviaQuizHelper.getAllOfTheQuestions().size() == 0) {
//If not added then add the ques,options in table
triviaQuizHelper.allQuestion();
}
//This will return us a list of data type TriviaQuestion
list = triviaQuizHelper.getAllOfTheQuestions();
//Now we gonna shuffle the elements of the list so that we will get questions randomly
Collections.shuffle(list);
//currentQuestion will hold the que, 4 option and ans for particular id
currentQuestion = list.get(qid);
//countDownTimer
countDownTimer = new CountDownTimer(22000, 1000) {
public void onTick(long millisUntilFinished) {
//here you can have your logic to set text to timeText
timeText.setText(String.valueOf(timeValue) + "\"");
//With each iteration decrement the time by 1 sec
timeValue -= 1;
//This means the user is out of time so onFinished will called after this iteration
if (timeValue == -1) {
//Since user is out of time setText as time up
resultText.setText(getString(R.string.timeup));
//Since user is out of time he won't be able to click any buttons
//therefore we will disable all four options buttons using this method
disableButton();
}
}
//Now user is out of time
public void onFinish() {
//We will navigate him to the time up activity using below method
timeUp();
}
}.start();
//This method will set the que and four options
updateQueAndOptions();
}
public void updateQueAndOptions() {
//This method will setText for que and options
questionText.setText(currentQuestion.getQuestion());
buttonA.setText(currentQuestion.getOptA());
buttonB.setText(currentQuestion.getOptB());
buttonC.setText(currentQuestion.getOptC());
buttonD.setText(currentQuestion.getOptD());
timeValue = 20;
//Now since the user has ans correct just reset timer back for another que- by cancel and start
countDownTimer.cancel();
countDownTimer.start();
//set the value of coin text
coinText.setText(String.valueOf(coinValue));
//Now since user has ans correct increment the coinvalue
coinValue++;
}
//Onclick listener for first button
public void buttonA(View view) {
//compare the option with the ans if yes then make button color green
if (currentQuestion.getOptA().equals(currentQuestion.getAnswer())) {
buttonA.setButtonColor(ContextCompat.getColor(getApplicationContext(),R.color.lightGreen));
//Check if user has not exceeds the que limit
if (qid < list.size() - 1) {
//Now disable all the option button since user ans is correct so
//user won't be able to press another option button after pressing one button
disableButton();
//Show the dialog that ans is correct
correctDialog();
}
//If user has exceeds the que limit just navigate him to GameWon activity
else {
gameWon();
}
}
//User ans is wrong then just navigate him to the PlayAgain activity
else {
gameLostPlayAgain();
}
}
//Onclick listener for sec button
public void buttonB(View view) {
if (currentQuestion.getOptB().equals(currentQuestion.getAnswer())) {
buttonB.setButtonColor(ContextCompat.getColor(getApplicationContext(),R.color.lightGreen));
if (qid < list.size() - 1) {
disableButton();
correctDialog();
} else {
gameWon();
}
} else {
gameLostPlayAgain();
}
}
//Onclick listener for third button
public void buttonC(View view) {
if (currentQuestion.getOptC().equals(currentQuestion.getAnswer())) {
buttonC.setButtonColor(ContextCompat.getColor(getApplicationContext(),R.color.lightGreen));
if (qid < list.size() - 1) {
disableButton();
correctDialog();
} else {
gameWon();
}
} else {
gameLostPlayAgain();
}
}
//Onclick listener for fourth button
public void buttonD(View view) {
if (currentQuestion.getOptD().equals(currentQuestion.getAnswer())) {
buttonD.setButtonColor(ContextCompat.getColor(getApplicationContext(),R.color.lightGreen));
if (qid < list.size() - 1) {
disableButton();
correctDialog();
} else {
gameWon();
}
} else {
gameLostPlayAgain();
}
}
//This method will navigate from current activity to GameWon
public void gameWon() {
Intent intent = new Intent(this, GameWon.class);
startActivity(intent);
finish();
}
//This method is called when user ans is wrong
//this method will navigate user to the activity PlayAgain
public void gameLostPlayAgain() {
Intent intent = new Intent(this, PlayAgain.class);
startActivity(intent);
finish();
}
//This method is called when time is up
//this method will navigate user to the activity Time_Up
public void timeUp() {
Intent intent = new Intent(this, Time_Up.class);
startActivity(intent);
finish();
}
//If user press home button and come in the game from memory then this
//method will continue the timer from the previous time it left
@Override
protected void onRestart() {
super.onRestart();
countDownTimer.start();
}
//When activity is destroyed then this will cancel the timer
@Override
protected void onStop() {
super.onStop();
countDownTimer.cancel();
}
//This will pause the time
@Override
protected void onPause() {
super.onPause();
countDownTimer.cancel();
}
//On BackPressed
@Override
public void onBackPressed() {
Intent intent = new Intent(this, HomeScreen.class);
startActivity(intent);
finish();
}
//This dialog is show to the user after he ans correct
public void correctDialog() {
final Dialog dialogCorrect = new Dialog(MainGameActivity.this);
dialogCorrect.requestWindowFeature(Window.FEATURE_NO_TITLE);
if (dialogCorrect.getWindow() != null) {
ColorDrawable colorDrawable = new ColorDrawable(Color.TRANSPARENT);
dialogCorrect.getWindow().setBackgroundDrawable(colorDrawable);
}
dialogCorrect.setContentView(R.layout.dialog_correct);
dialogCorrect.setCancelable(false);
dialogCorrect.show();
//Since the dialog is show to user just pause the timer in background
onPause();
TextView correctText = (TextView) dialogCorrect.findViewById(R.id.correctText);
FButton buttonNext = (FButton) dialogCorrect.findViewById(R.id.dialogNext);
//Setting type faces
correctText.setTypeface(sb);
buttonNext.setTypeface(sb);
//OnCLick listener to go next que
buttonNext.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
//This will dismiss the dialog
dialogCorrect.dismiss();
//it will increment the question number
qid++;
//get the que and 4 option and store in the currentQuestion
currentQuestion = list.get(qid);
//Now this method will set the new que and 4 options
updateQueAndOptions();
//reset the color of buttons back to white
resetColor();
//Enable button - remember we had disable them when user ans was correct in there particular button methods
enableButton();
}
});
}
//This method will make button color white again since our one button color was turned green
public void resetColor() {
buttonA.setButtonColor(ContextCompat.getColor(getApplicationContext(),R.color.white));
buttonB.setButtonColor(ContextCompat.getColor(getApplicationContext(),R.color.white));
buttonC.setButtonColor(ContextCompat.getColor(getApplicationContext(),R.color.white));
buttonD.setButtonColor(ContextCompat.getColor(getApplicationContext(),R.color.white));
}
//This method will disable all the option button
public void disableButton() {
buttonA.setEnabled(false);
buttonB.setEnabled(false);
buttonC.setEnabled(false);
buttonD.setEnabled(false);
}
//This method will all enable the option buttons
public void enableButton() {
buttonA.setEnabled(true);
buttonB.setEnabled(true);
buttonC.setEnabled(true);
buttonD.setEnabled(true);
}
}
PlayAgain.java
package sarveshchavan777.triviaquiz;
import android.app.Activity;
import android.content.Intent;
import android.graphics.Typeface;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
public class PlayAgain extends Activity {
Button playAgain;
TextView wrongAnsText;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.play_again);
//Initialize
playAgain = (Button) findViewById(R.id.playAgainButton);
wrongAnsText = (TextView)findViewById(R.id.wrongAns);
//play again button onclick listener
playAgain.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
Intent intent = new Intent(PlayAgain.this, MainGameActivity.class);
startActivity(intent);
finish();
}
});
//Setting typefaces for textview and button - this will give stylish fonts on textview and button
Typeface typeface = Typeface.createFromAsset(getAssets(),"fonts/shablagooital.ttf");
playAgain.setTypeface(typeface);
wrongAnsText.setTypeface(typeface);
}
@Override
public void onBackPressed() {
finish();
}
}
Time_Up.java
package sarveshchavan777.triviaquiz;
import android.content.Intent;
import android.graphics.Typeface;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.TextView;
import info.hoang8f.widget.FButton;
public class Time_Up extends AppCompatActivity {
FButton playAgainButton;
TextView timeUpText;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_time__up);
//Initialize
playAgainButton = (FButton)findViewById(R.id.playAgainButton);
timeUpText = (TextView)findViewById(R.id.timeUpText);
//play again button onclick listener
playAgainButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
Intent intent = new Intent(Time_Up.this,MainGameActivity.class);
startActivity(intent);
finish();
}
});
//Setting typefaces for textview and button - this will give stylish fonts on textview and button
Typeface typeface = Typeface.createFromAsset(getAssets(),"fonts/shablagooital.ttf");
timeUpText.setTypeface(typeface);
playAgainButton.setTypeface(typeface);
}
@Override
public void onBackPressed() {
super.onBackPressed();
finish();
}
}
TriviaQuestion.java
package sarveshchavan777.triviaquiz;
import android.app.Activity;
public class TriviaQuestion extends Activity {
private int id;
private String question;
private String opta;
private String optb;
private String optc;
private String optd;
private String answer;
public TriviaQuestion(String q, String oa, String ob, String oc, String od, String ans) {
question = q;
opta = oa;
optb = ob;
optc = oc;
optd = od;
answer = ans;
}
public TriviaQuestion() {
id = 0;
question = "";
opta = "";
optb = "";
optc = "";
optd = "";
answer = "";
}
public String getQuestion() {
return question;
}
public String getOptA() {
return opta;
}
public String getOptB() {
return optb;
}
public String getOptC() {
return optc;
}
public String getOptD() {
return optd;
}
public String getAnswer() {
return answer;
}
public void setId(int i) {
id = i;
}
public void setQuestion(String q1) {
question = q1;
}
public void setOptA(String o1) {
opta = o1;
}
public void setOptB(String o2) {
optb = o2;
}
public void setOptC(String o3) {
optc = o3;
}
public void setOptD(String o4) {
optd = o4;
}
public void setAnswer(String ans) {
answer = ans;
}
}
TriviaQuizHelper.java
package sarveshchavan777.triviaquiz;
import android.content.ContentValues;
import android.content.Context;
import android.database.Cursor;
import android.database.sqlite.SQLiteDatabase;
import android.database.sqlite.SQLiteOpenHelper;
import java.util.ArrayList;
import java.util.List;
class TriviaQuizHelper extends SQLiteOpenHelper {
private Context context;
private static final String DB_NAME = "TQuiz.db";
//If you want to add more questions or wanna update table values
//or any kind of modification in db just increment version no.
private static final int DB_VERSION = 3;
//Table name
private static final String TABLE_NAME = "TQ";
//Id of question
private static final String UID = "_UID";
//Question
private static final String QUESTION = "QUESTION";
//Option A
private static final String OPTA = "OPTA";
//Option B
private static final String OPTB = "OPTB";
//Option C
private static final String OPTC = "OPTC";
//Option D
private static final String OPTD = "OPTD";
//Answer
private static final String ANSWER = "ANSWER";
//So basically we are now creating table with first column-id , sec column-question , third column -option A, fourth column -option B , Fifth column -option C , sixth column -option D , seventh column - answer(i.e ans of question)
private static final String CREATE_TABLE = "CREATE TABLE " + TABLE_NAME + " ( " + UID + " INTEGER PRIMARY KEY AUTOINCREMENT , " + QUESTION + " VARCHAR(255), " + OPTA + " VARCHAR(255), " + OPTB + " VARCHAR(255), " + OPTC + " VARCHAR(255), " + OPTD + " VARCHAR(255), " + ANSWER + " VARCHAR(255));";
//Drop table query
private static final String DROP_TABLE = "DROP TABLE IF EXISTS " + TABLE_NAME;
TriviaQuizHelper(Context context) {
super(context, DB_NAME, null, DB_VERSION);
this.context = context;
}
@Override
public void onCreate(SQLiteDatabase sqLiteDatabase) {
//OnCreate is called only once
sqLiteDatabase.execSQL(CREATE_TABLE);
}
@Override
public void onUpgrade(SQLiteDatabase sqLiteDatabase, int i, int i1) {
//OnUpgrade is called when ever we upgrade or increment our database version no
sqLiteDatabase.execSQL(DROP_TABLE);
onCreate(sqLiteDatabase);
}
void allQuestion() {
ArrayList<TriviaQuestion> arraylist = new ArrayList<>();
arraylist.add(new TriviaQuestion("Galileo was an Italian astronomer who developed?", "Telescope", "Airoplane", "Electricity", "Train", "Telescope"));
arraylist.add(new TriviaQuestion("Who is the father of Geometry ?", "Aristotle", "Euclid", "Pythagoras", "Kepler", "Euclid"));
arraylist.add(new TriviaQuestion("Who was known as Iron man of India ?", "Govind Ballabh Pant", "Jawaharlal Nehru", "Subhash Chandra Bose", "Sardar Vallabhbhai Patel", "Sardar Vallabhbhai Patel"));
arraylist.add(new TriviaQuestion("The first woman in space was ?", "Valentina Tereshkova", "Sally Ride", "Naidia Comenci", "Tamara Press", "Valentina Tereshkova"));
arraylist.add(new TriviaQuestion("Who is the Flying Sikh of India ?", "Mohinder Singh", "Joginder Singh", "Ajit Pal Singh", "Milkha singh", "Milkha singh"));
arraylist.add(new TriviaQuestion("The Indian to beat the computers in mathematical wizardry is", "Ramanujam", "Rina Panigrahi", "Raja Ramanna", "Shakunthala Devi", "Shakunthala Devi"));
arraylist.add(new TriviaQuestion("Who is Larry Pressler ?", "Politician", "Painter", "Actor", "Tennis player", "Politician"));
arraylist.add(new TriviaQuestion("Michael Jackson is a distinguished person in the field of ?", "Pop Music", "Jounalism", "Sports", "Acting", "Pop Music"));
arraylist.add(new TriviaQuestion("The first Indian to swim across English channel was ?", "V. Merchant", "P. K. Banerji", "Mihir Sen", "Arati Saha", "Mihir Sen"));
arraylist.add(new TriviaQuestion("Who was the first Indian to make a movie?", "Dhundiraj Govind Phalke", " Asha Bhonsle", " Ardeshir Irani", "V. Shantaram", "Dhundiraj Govind Phalke"));
arraylist.add(new TriviaQuestion("Who is known as the ' Saint of the gutters ?", "B.R.Ambedkar", "Mother Teresa", "Mahatma Gandhi", "Baba Amte", "Mother Teresa"));
arraylist.add(new TriviaQuestion("Who invented the famous formula E=mc^2", "Albert Einstein", "Galilio", "Sarvesh", "Bill Gates", "Albert Einstein"));
arraylist.add(new TriviaQuestion("Who is elected as president of us 2016", "Donald Trump", "Hilary Clinton", "Jhon pol", "Barack Obama", "Donald Trump"));
arraylist.add(new TriviaQuestion("Who was the founder of company Microsoft", "Bill Gates", "Bill Clinton", "Jhon rio", "Steve jobs", "Bill Gates"));
arraylist.add(new TriviaQuestion("Who was the founder of company Apple ?", "Steve Jobs", "Steve Washinton", "Bill Gates", "Jobs Wills", "Steve Jobs"));
arraylist.add(new TriviaQuestion("Who was the founder of company Google ?", "Steve Jobs", "Bill Gates", "Larry Page", "Sundar Pichai", "Larry Page"));
arraylist.add(new TriviaQuestion("Who is know as god of cricket ?", "Sachin Tendulkar", "Kapil Dev", "Virat Koli", "Dhoni", "Sachin Tendulkar"));
arraylist.add(new TriviaQuestion("who has won ballon d'or of 2015 ?", "Lionel Messi", "Cristiano Ronaldo", "Neymar", "Kaka", "Lionel Messi"));
arraylist.add(new TriviaQuestion("who has won ballon d'or of 2014 ?", "Neymar", "Lionel Messi", "Cristiano Ronaldo", "Kaka", "Cristiano Ronaldo"));
arraylist.add(new TriviaQuestion("the Founder of the most famous gaming platform steam is ?", "Bill Cliton", "Bill Williams", "Gabe Newell", "Bill Gates", "Gabe Newell"));
this.addAllQuestions(arraylist);
}
private void addAllQuestions(ArrayList<TriviaQuestion> allQuestions) {
SQLiteDatabase db = this.getWritableDatabase();
db.beginTransaction();
try {
ContentValues values = new ContentValues();
for (TriviaQuestion question : allQuestions) {
values.put(QUESTION, question.getQuestion());
values.put(OPTA, question.getOptA());
values.put(OPTB, question.getOptB());
values.put(OPTC, question.getOptC());
values.put(OPTD, question.getOptD());
values.put(ANSWER, question.getAnswer());
db.insert(TABLE_NAME, null, values);
}
db.setTransactionSuccessful();
} finally {
db.endTransaction();
db.close();
}
}
List<TriviaQuestion> getAllOfTheQuestions() {
List<TriviaQuestion> questionsList = new ArrayList<>();
SQLiteDatabase db = this.getWritableDatabase();
db.beginTransaction();
String coloumn[] = {UID, QUESTION, OPTA, OPTB, OPTC, OPTD, ANSWER};
Cursor cursor = db.query(TABLE_NAME, coloumn, null, null, null, null, null);
while (cursor.moveToNext()) {
TriviaQuestion question = new TriviaQuestion();
question.setId(cursor.getInt(0));
question.setQuestion(cursor.getString(1));
question.setOptA(cursor.getString(2));
question.setOptB(cursor.getString(3));
question.setOptC(cursor.getString(4));
question.setOptD(cursor.getString(5));
question.setAnswer(cursor.getString(6));
questionsList.add(question);
}
db.setTransactionSuccessful();
db.endTransaction();
cursor.close();
db.close();
return questionsList;
}
}